What is cascading and different types?
Cascading in Hibernate is a feature that allows you to propagate operations from a parent entity to its associated child entities. This means that if you perform an operation (like persist, delete, or update) on a parent entity, the same operation can be automatically applied to its related child entities. Cascading is useful for maintaining the integrity and consistency of related data without requiring explicit operations on each entity.
Types of Cascading
- CascadeType.PERSIST : Propagates the persist operation from the parent entity to the child entities.
- CascadeType.MERGE : Propagates the merge operation from the parent entity to the child entities.
- CascadeType.REMOVE : Propagates the remove operation from the parent entity to the child entities.
- CascadeType.REFRESH : Propagates the refresh operation from the parent entity to the child entities.
- CascadeType.DETACH : Propagates the detach operation from the parent entity to the child entities.
- CascadeType.ALL : Propagates all operations (persist, merge, remove, refresh, detach) from the parent entity to the child entities.
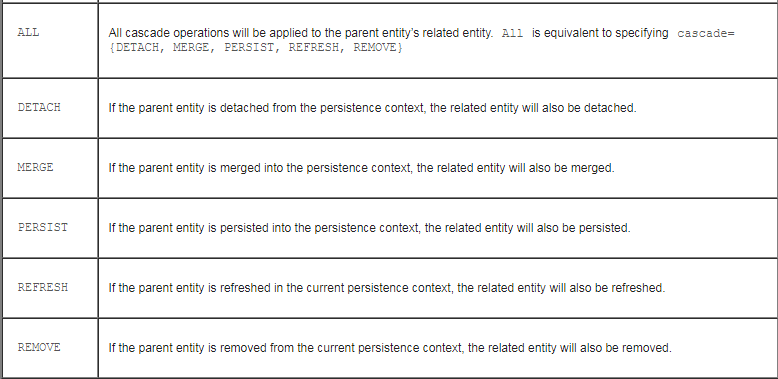
Table of Contents
Java Example
Let’s demonstrate cascading with an example involving Author and Book entities, where an author can have multiple books.
Step 1: Define the Entities
Step 1: Define the Entities
Author.java
java
import javax.persistence.*;
import java.util.ArrayList;
import java.util.List;
@Entity
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(mappedBy = "author", cascade = CascadeType.ALL, orphanRemoval = true)
private List<Book> books = new ArrayList<>();
// Getters and setters
public void addBook(Book book) {
books.add(book);
book.setAuthor(this);
}
public void removeBook(Book book) {
books.remove(book);
book.setAuthor(null);
}
}
Book.java
java
import javax.persistence.*;
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
@ManyToOne
@JoinColumn(name = "author_id")
private Author author;
// Getters and setters
}
Step 2: Configure Hibernate
hibernate.cfg.xml
xml
<!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<!-- Database connection settings -->
<property name="hibernate.connection.driver_class">com.mysql.cj.jdbc.Driver</property>
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/mydb</property>
<property name="hibernate.connection.username">root</property>
<property name="hibernate.connection.password">password</property>
<!-- JDBC connection pool settings ... using built-in test pool -->
<property name="hibernate.c3p0.min_size">5</property>
<property name="hibernate.c3p0.max_size">20</property>
<property name="hibernate.c3p0.timeout">300</property>
<property name="hibernate.c3p0.max_statements">50</property>
<property name="hibernate.c3p0.idle_test_period">3000</property>
<!-- Specify dialect -->
<property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property>
<!-- Update the database schema on startup -->
<property name="hibernate.hbm2ddl.auto">update</property>
<!-- Mappings -->
<mapping class="com.example.Author"/>
<mapping class="com.example.Book"/>
</session-factory>
</hibernate-configuration>
Step 3: Main Application
Main.java
java
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class Main {
public static void main(String[] args) {
// Set up the Hibernate session factory
SessionFactory sessionFactory = new Configuration().configure().buildSessionFactory();
Session session = null;
try {
session = sessionFactory.openSession();
session.beginTransaction();
// Create an author
Author author = new Author();
author.setName("J.K. Rowling");
// Create books and add them to the author
Book book1 = new Book();
book1.setTitle("Harry Potter and the Philosopher's Stone");
author.addBook(book1);
Book book2 = new Book();
book2.setTitle("Harry Potter and the Chamber of Secrets");
author.addBook(book2);
// Save the author (will cascade to save books)
session.save(author);
// Commit the transaction
session.getTransaction().commit();
} catch (Exception e) {
if (session != null) {
session.getTransaction().rollback();
}
e.printStackTrace();
} finally {
if (session != null) {
session.close();
}
sessionFactory.close();
}
}
}
Explanation of the Example
- 1. Â Define the Entities :
- Â Author entity has a OneToMany relationship with the Book entity and uses CascadeType.ALL to propagate all operations.
- Book entity has a ManyToOne relationship with the Author entity.
- 2. Â Configure Hibernate : The hibernate.cfg.xml file configures Hibernate to connect to a MySQL database and maps the Author and Book entities.
- 3. Â Main Application :
- We open a session and begin a transaction.
- Create an Author and add Book entities to it.
- Save the Author, which cascades the save operation to the Book entities.
- Commit the transaction if successful; otherwise, rollback in case of an exception.
- This example demonstrates the use of cascading to simplify the management of related entities in Hibernate.