Use @SessionAttributes in Spring MVC
@SessionAttributes is an annotation in Spring MVC used to store model attributes in the HTTP session. It allows you to keep certain data across multiple requests within a user’s session. This can be useful for scenarios like maintaining user data or form data throughout the user’s interaction with the web application.
Purpose:
It helps in retaining the state of certain attributes between different requests within the same session.
Steps to Use `@SessionAttributes`:
- Annotate the Controller: Use `@SessionAttributes` to specify which attributes should be stored in the session.
- Add Attributes to the Model: Ensure that the attributes defined in `@SessionAttributes` are added to the model.
- Retrieve and Use Attributes: Access these session attributes in different request handlers.
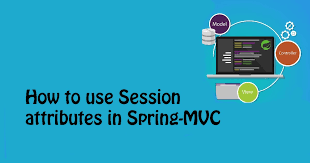
Table of Contents
Example
1. Annotate the Controller
Use `@SessionAttributes` to specify which attributes should be stored in the session.
UserController.java
```java
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.SessionAttributes;
@Controller
@SessionAttributes("user")
public class UserController {
@ModelAttribute("user")
public User createUser() {
// Create a new User object and add it to the model
return new User();
}
@RequestMapping(value = "/showForm", method = RequestMethod.GET)
public String showForm(Model model) {
// No need to explicitly add the User object to the model
return "userForm";
}
@RequestMapping(value = "/processForm", method = RequestMethod.POST)
public String processForm(@ModelAttribute("user") User user, Model model) {
// Process the form and the User object
model.addAttribute("user", user);
return "userResult";
}
}
```
In this example:
@SessionAttributes("user")
: Specifies that theuser
attribute should be stored in the session.@ModelAttribute("user")
: Creates aUser
object and adds it to the model, which will be stored in the session.
2. Add Attributes to the Model
The User
object is automatically added to the session when the form is displayed or processed, as specified by the @ModelAttribute
annotation.
userForm.jsp
```jsp
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<html>
<head>
<title>User Form</title>
</head>
<body>
<form action="processForm" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name" value="${user.name}"/>
<label for="email">Email:</label>
<input type="text" id="email" name="email" value="${user.email}"/>
<button type="submit">Submit</button>
</form>
</body>
</html>
```
userResult.jsp
```jsp
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<html>
<head>
<title>User Result</title>
</head>
<body>
<h2>User Information</h2>
<p>Name: ${user.name}</p>
<p>Email: ${user.email}</p>
</body>
</html>
```
In this example:
userForm.jsp
: Displays the form where the user can input their data. The form fields are pre-populated with values from theuser
attribute.userResult.jsp
: Displays the submitted user information.
3. Retrieve and Use Attributes
Attributes stored in the session can be accessed across different requests. When you process the form submission, the user
attribute is available for further use.
User.java
```java
public class User {
private String name;
private String email;
// Getters and Setters
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public String getEmail() { return email; }
public void setEmail(String email) { this.email = email; }
}
```
In this example:
User
: Represents the data model that is stored in the session.
Summary
@SessionAttributes
: Stores specified attributes in the HTTP session for persistence across multiple requests.
Steps:
- Annotate Controller: Use
@SessionAttributes
to specify session attributes. - Add Attributes: Add attributes to the model using
@ModelAttribute
. - Retrieve Attributes: Access session attributes in different requests.