Read data from the FORM in Spring MVC
In Spring MVC, you can read data from HTML forms using several methods, each suited for different scenarios. The primary methods are:
1. Using `@RequestParam`:
- Definition: The `@RequestParam` annotation binds request parameters (query parameters, form parameters) to method parameters in a controller. It is commonly used for simple form submissions.
- Purpose: To extract individual form fields from the HTTP request.
2. Using `@ModelAttribute`:
- Definition: The `@ModelAttribute` annotation binds form data to a model object. It allows you to automatically populate an object with form data.
- Purpose: To bind multiple form fields to a single object, making it easier to handle complex forms.
3. Using `HttpServletRequest`:
- Definition: The `HttpServletRequest` object provides access to all request parameters and attributes.
- Â Purpose: To manually retrieve form data when you need more control or when dealing with dynamic parameter names.
4. Using `@RequestBody`:
- Definition: The `@RequestBody` annotation is used for reading data from the request body. It is often used with JSON or XML data.
- Purpose: To handle form data sent as JSON or other formats in the request body.
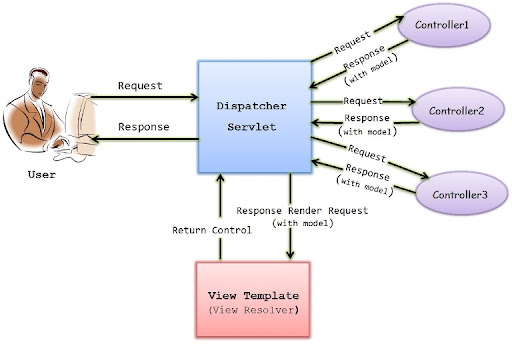
Table of Contents
1. Using @RequestParam
Explanation:
- Purpose: Extract individual parameters from the form submission.
Using
@RequestParam
UserController.java
```java
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@GetMapping("/submitForm")
public String submitForm(@RequestParam("name") String name, @RequestParam("email") String email) {
return "Received name: " + name + ", email: " + email;
}
}
```
In this example:
@RequestParam("name")
and@RequestParam("email")
: Extractname
andemail
parameters from the form.
2. Using `@ModelAttribute`
Explanation: Purpose: Bind form data to a model object.
Example
Example:
User.java
```java
public class User {
private String name;
private String email;
// Getters and Setters
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public String getEmail() { return email; }
public void setEmail(String email) { this.email = email; }
}
```
UserController.java
```java
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class UserController {
@RequestMapping("/showForm")
public String showForm() {
return "userForm";
}
@PostMapping("/processForm")
public String processForm(@ModelAttribute User user) {
return "Processed user with name: " + user.getName() + " and email: " + user.getEmail();
}
}
```
userForm.jsp
```jsp
<html>
<head>
<title>User Form</title>
</head>
<body>
<form action="processForm" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name"/>
<label for="email">Email:</label>
<input type="text" id="email" name="email"/>
<button type="submit">Submit</button>
</form>
</body>
</html>
```
In this example:
@ModelAttribute
: Binds form data to theUser
object.
3. Using `HttpServletRequest`
Explanation:
Purpose: Manually retrieve form data using `HttpServletRequest`.
Example
UserController.java
```java
import javax.servlet.http.HttpServletRequest;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@PostMapping("/processForm")
public String processForm(HttpServletRequest request) {
String name = request.getParameter("name");
String email = request.getParameter("email");
return "Received name: " + name + ", email: " + email;
}
}
```
In this example:
request.getParameter("name")
andrequest.getParameter("email")
: Manually retrieve parameters from the request.
4. Using @RequestBody
Explanation:
- Purpose: Read data from the request body, commonly used with JSON data.
Example
User.java
```java
public class User {
private String name;
private String email;
// Getters and Setters
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public String getEmail() { return email; }
public void setEmail(String email) { this.email = email; }
}
```
UserController.java
```java
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@PostMapping("/submitJson")
public String submitJson(@RequestBody User user) {
return "Received user with name: " + user.getName() + " and email: " + user.getEmail();
}
}
```
In this example:
@RequestBody
: Binds the JSON data from the request body to theUser
object.
Summary
@RequestParam
: For extracting individual request parameters.@ModelAttribute
: For binding form data to a model object.HttpServletRequest
: For manually retrieving parameters from the request.@RequestBody
: For reading data from the request body, typically used with JSON.