Publishing an Android app on the Google Play
Publishing an Android app on the Google Play Store involves several steps, from preparing the app for release to actually submitting it for review and distribution. This process ensures that your app is properly configured, tested, and compliant with Google’s policies.
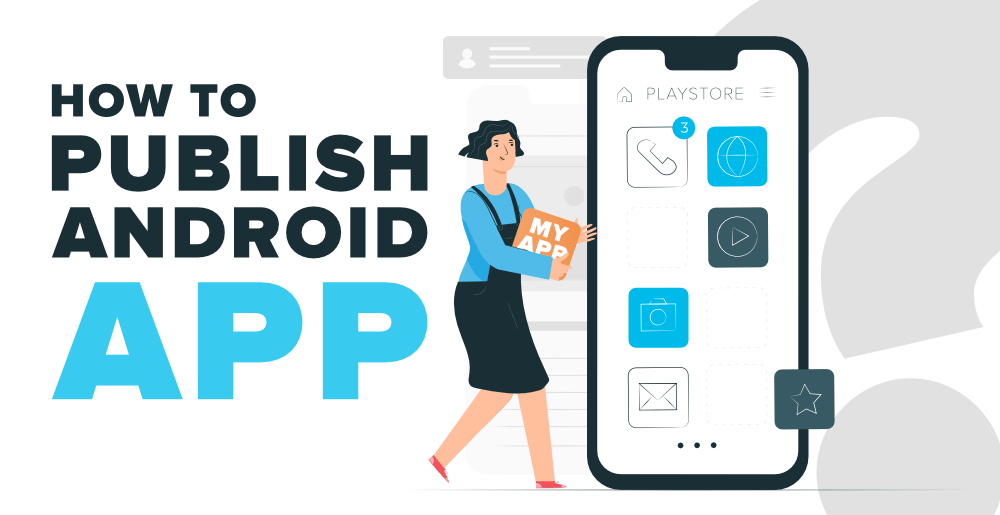
Table of Contents
Here’s a step-by-step breakdown of the process
1. Prepare Your App for Release
Remove Debug Code: Ensure that all debug code (like `Log.d`) is removed or disable. Set Version Information: In your `build.gradle` file, make sure to properly set `versionCode` and `versionName`. These fields help manage updates to your app. Publishing Sign Your App: You must sign your app with a release key. Android apps require signing for identification. You can either use the Google Play App Signing service or manage the signing keys yourself.
2. Generate the Signed APK or AAB
You need to build a signed APK or Android App Bundle (AAB) for distribution. AAB is preferred because it optimizes the app size based on the user’s device. Go to Build > Generate Signed Bundle / APK in Android Studio, select your signing key, and generate the release build.
3. Create a Developer Account
If you don’t already have one, you’ll need to create a Google Play Developer account. The one-time registration fee is $25.
4. Create a New Application in the Google Play Console:
Go to the [Google Play Console](https://play.google.com/console), sign in with your developer account, and create a new application. You’ll be prompted to enter basic details like the app name, language, and description.
5. Provide Store Listing Information:
Title and Description: Write a concise and informative title and description. Screenshots and Promo Graphics: Upload screenshots of your app in action, a high-res icon (512×512), a feature graphic (1024×500), and any promotional videos if available.
- Category and Tags: Choose the appropriate category (e.g., Game, Productivity) and relevant tags.
- Content Rating: Fill out the content rating questionnaire to ensure your app is rated appropriately.
6. Set Pricing and Distribution
- Decide if your app will be free or paid. Once published as free, you cannot change it to paid.
- Select the countries where your app will be available.
- Set up distribution channels like phones, tablets, and other supported devices.
7. Prepare and Submit the Release:
Upload your signed APK or AAB file. Add release notes and track details (e.g., production, beta, or alpha). Ensure you’ve set up any necessary testing (internal, closed, or open testing) before going live.
8. Review and Publish
Before publishing, the Play Console will check for policy compliance and any issues. Once everything is set, click “Publish” to submit your app for review. The review process can take a few hours to several days.
9. Monitor and Update Your App
Once published, monitor your app’s performance, user feedback, and crashes using the Play Console. Regularly update your app with new features, bug fixes, and improvements.
Java Example
Here’s a basic overview of some of the steps involved in preparing your app for release using Java and Android Studio:
1. Setting the Version Code and Name in `build.gradle`:
```groovy
android {
compileSdkVersion 33
defaultConfig {
applicationId "com.example.myapp"
minSdkVersion 21
targetSdkVersion 33
versionCode 1
versionName "1.0"
}
...
}
```
2. Signing the App Programmatically (If Needed):
```java
// Typically, signing is handled through the Gradle build script.
// Here’s a sample configuration for signing:
signingConfigs {
release {
storeFile file("my-release-key.jks")
storePassword "password"
keyAlias "my-alias"
keyPassword "key-password"
}
}
buildTypes {
release {
signingConfig signingConfigs.release
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
```
This configuration is essential for producing a release build that is signed and ready for distribution.
3. Generating the Release Build in Android Studio:
- In Android Studio, navigate to Build > Generate Signed Bundle / APK. Select either Android App Bundle or APK, then follow the wizard to create a signed release version.
4. Uploading the APK/AAB to the Google Play Console:
- Once generated, you upload the APK/AAB file through the Play Console, as explained in the process above.