list of all beans Spring boot application
In a Spring Boot application, you may sometimes need to retrieve and inspect the list of all beans managed by the Spring container. This can be useful for debugging, testing, or understanding the application’s context. You can achieve this using the `ApplicationContext` which holds all the bean definitions and their instances.
Key Methods to Get Beans:
- Using `ApplicationContext.getBeansOfType(Class<T> type)`: Retrieves all beans of a specific type.
- Using `ApplicationContext.getBeanDefinitionNames()`: Lists all bean names defined in the application context.
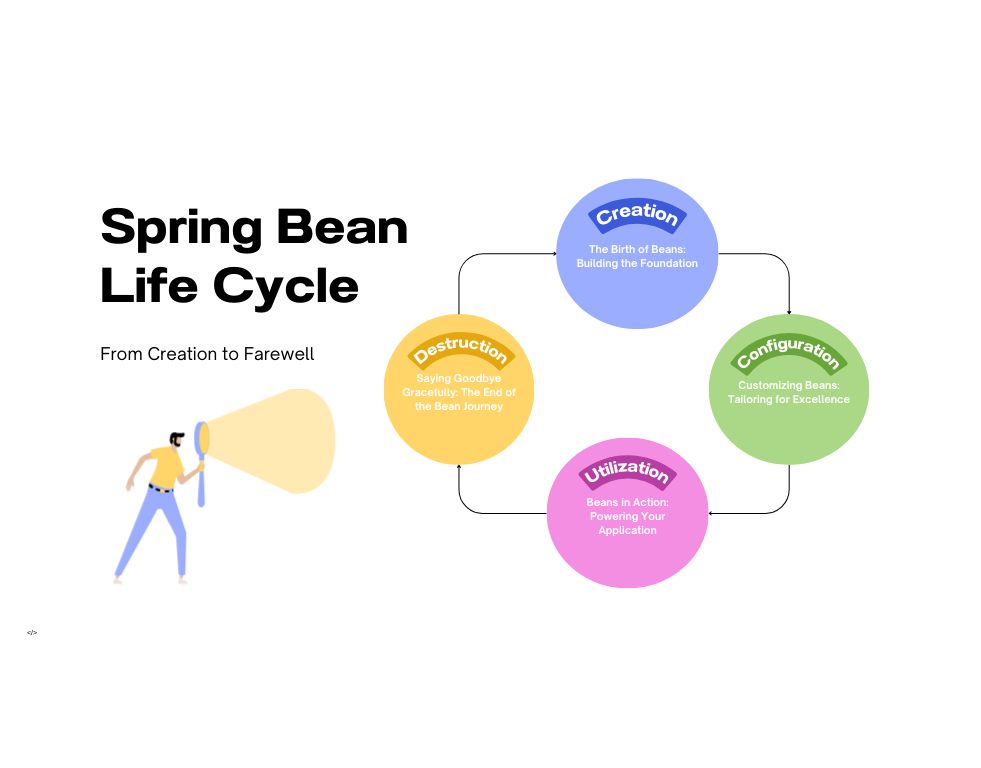
Table of Contents
”1.
Example:
To get all beans of a specific type, such as `MyService`, you can use `ApplicationContext.getBeansOfType()`.
Define a Service Class:
```java
package com.example.demo.service;
import org.springframework.stereotype.Service;
@Service
public class MyService {
public String getName() {
return "MyService";
}
}
```
Define Another Service Class:
```java
package com.example.demo.service;
import org.springframework.stereotype.Service;
@Service
public class AnotherService {
public String getName() {
return "AnotherService";
}
}
```
Access Beans in a Component:
```java
package com.example.demo.component;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.stereotype.Component;
import javax.annotation.PostConstruct;
@Component
public class BeanLister {
@Autowired
private ApplicationContext applicationContext;
@PostConstruct
public void listBeans() {
// Get all beans of type MyService
Map<String, MyService> beans = applicationContext.getBeansOfType(MyService.class);
beans.forEach((name, bean) -> {
System.out.println("Bean name: " + name + ", Bean: " + bean.getName());
});
}
}
```
Explanation
@Autowired
: Injects theApplicationContext
into the component.applicationContext.getBeansOfType(MyService.class)
: Retrieves all beans of typeMyService
and prints their names.
2. Using
ApplicationContext.getBeanDefinitionNames()
Example:
To list all bean names defined in the application context:
Access Beans in a Component:
```java
package com.example.demo.component;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.stereotype.Component;
import javax.annotation.PostConstruct;
@Component
public class BeanLister {
@Autowired
private ApplicationContext applicationContext;
@PostConstruct
public void listAllBeanNames() {
// Get all bean names
String[] beanNames = applicationContext.getBeanDefinitionNames();
for (String beanName : beanNames) {
System.out.println("Bean name: " + beanName);
}
}
}
```
Explanation:
applicationContext.getBeanDefinitionNames()
: Retrieves all bean names and prints them.
Conclusion list of all beans Spring boot application
- Get Beans of a Specific Type:
- Method: Use
applicationContext.getBeansOfType(Class<T> type)
. - Example: Retrieves and lists all beans of type
MyService
.
- Method: Use
- List All Bean Names:
- Method: Use
applicationContext.getBeanDefinitionNames()
. - Example: Lists all bean names defined in the application context.
- Method: Use