Java Persistence API
Java Persistence API (JPA) is a specification for object-relational mapping (ORM) in Java, providing a standardized way to map Java objects to database tables and manage persistent data. JPA defines a set of interfaces and annotations that can be implemented by various ORM tools such as Hibernate, EclipseLink, and OpenJPA.
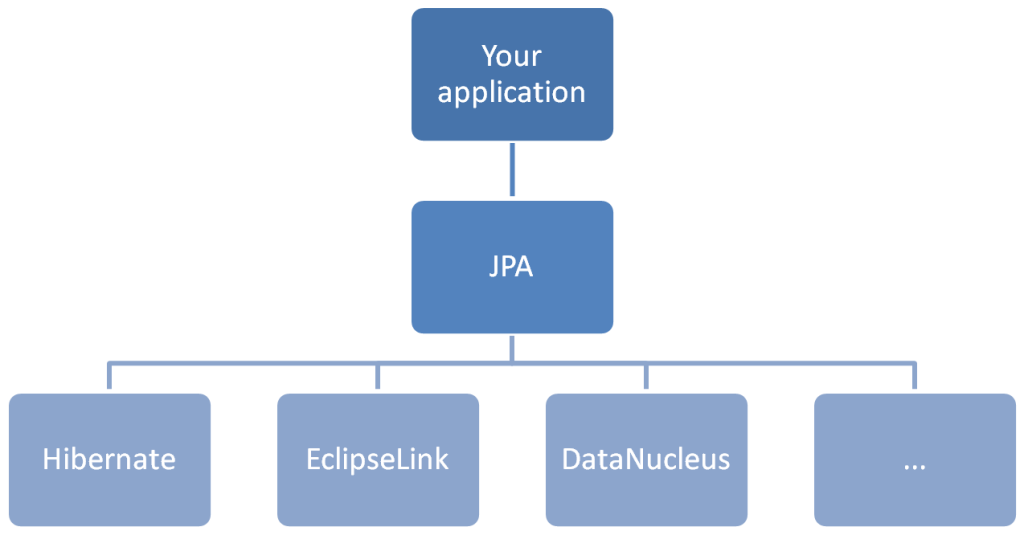
Table of Contents
Key Features of JPA:
- 1. Annotations for Mapping:
- JPA uses annotations to define how Java objects map to database tables, columns, and relationships.
- Examples include @Entity, @Table, @Id, @Column, and @OneToMany.
- 2. Entity Management:
- JPA manages the lifecycle of entities (Java objects mapped to database tables) through the EntityManager interface.
- Operations like persist, merge, remove, and find are performed using the EntityManager.
- 3. JPQL (Java Persistence Query Language):
- JPQL is a query language similar to SQL but operates on the entity object model rather than the relational database.
- It allows developers to write database queries using Java objects and properties.
- 4. Transaction Management:
- JPA provides support for managing transactions, ensuring that database operations are executed in a consistent and reliable manner.
- Annotations like @Transactional are used to define transactional boundaries.
- 5. Caching:
- JPA includes mechanisms for caching entities, which improves performance by reducing the number of database accesses.
- Second-level caching can be configured to store entities across multiple sessions.
Java Example
Let’s demonstrate a simple JPA application using Spring Boot and Hibernate as the JPA provider. This example will showcase how to define an entity, a repository, and perform basic CRUD operations.
Step 1: Add Dependencies
Add the following dependencies to your pom.xml file for a Spring Boot application:
xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
</dependencies>
Step 2: Define the Entity
Create a Book entity class:
java
package com.example.demo.model;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
private String author;
// Constructors, getters, and setters
public Book() {}
public Book(String title, String author) {
this.title = title;
this.author = author;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
}
Step 3: Define the Repository
Create a BookRepository interface:
java
package com.example.demo.repository;
import com.example.demo.model.Book;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface BookRepository extends JpaRepository<Book, Long> {
}
Step 4: Define the Service
Create a BookService class:
java
package com.example.demo.service;
import com.example.demo.model.Book;
import com.example.demo.repository.BookRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class BookService {
@Autowired
private BookRepository bookRepository;
public List<Book> getAllBooks() {
return bookRepository.findAll();
}
public Book getBookById(Long id) {
return bookRepository.findById(id).orElse(null);
}
public void saveBook(Book book) {
bookRepository.save(book);
}
public void deleteBook(Long id) {
bookRepository.deleteById(id);
}
}
Step 5: Define the Controller
Create a BookController class:
java
package com.example.demo.controller;
import com.example.demo.model.Book;
import com.example.demo.service.BookService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/api/books")
public class BookController {
@Autowired
private BookService bookService;
@GetMapping
public List<Book> getAllBooks() {
return bookService.getAllBooks();
}
@GetMapping("/{id}")
public Book getBookById(@PathVariable Long id) {
return bookService.getBookById(id);
}
@PostMapping
public void saveBook(@RequestBody Book book) {
bookService.saveBook(book);
}
@DeleteMapping("/{id}")
public void deleteBook(@PathVariable Long id) {
bookService.deleteBook(id);
}
}
Step 6: Application Properties
Configure the database in application.properties:
properties
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=password
spring.jpa.database-platform=org.hibernate.dialect.H2Dialect
spring.h2.console.enabled=true
Explanation of Example
- Book Entity (Book.java) :
- Annotated with @Entity to mark it as a JPA entity.
- Fields id, title, and author are mapped to the database table columns.
- @Id and @GeneratedValue annotations are used for the primary key generation.
- Book Repository (BookRepository.java) :
- Extends JpaRepository to provide CRUD operations on Book entities.
- Book Service (BookService.java) :
- Contains business logic for managing Book entities.
- Uses BookRepository to perform CRUD operations.
- Book Controller (BookController.java) :
- Defines RESTful endpoints to expose Book management functionality.
- Uses BookService to handle HTTP requests for retrieving, adding, and deleting books.
- Application Properties (application.properties) :
- Configures an in-memory H2 database for the application.
- Enables the H2 console for viewing the database schema and data.
Summary
JPA provides a standard way to map Java objects to database tables, manage entity lifecycle, execute queries, and handle transactions. Using JPA with Spring Boot and Hibernate simplifies the development of robust and maintainable data access layers in Java applications.