How does a spring application get started?
A Spring application typically goes through several steps to get started. These steps include setting up the Spring context, configuring beans, and starting the application. Here’s an overview of the process:
- Setup: Adding dependencies and creating the main application class.
- Configuration: Setting up application properties and configuration classes.
- Context Initialization: Initializing the Spring application context, which manages the beans and their lifecycle.
- Bean Creation: Creating and injecting beans based on configuration.
- Running the Application: The main method triggers the start of the application.
Steps to Start a Spring Application
- Add Dependencies: Include Spring Boot Starter dependencies in your `pom.xml` or `build.gradle` file.
- Create Main Application Class: Create a class with the `@SpringBootApplication` annotation and a `main` method to run the Spring application.
- Configure Application Properties: Use `application.properties` or `application.yml` to configure application settings.
- Create Configuration Classes: Define beans and other configurations using Java configuration classes annotated with `@Configuration`.
- Run the Application: The Spring Boot application is started by running the `main` method of the main application class.
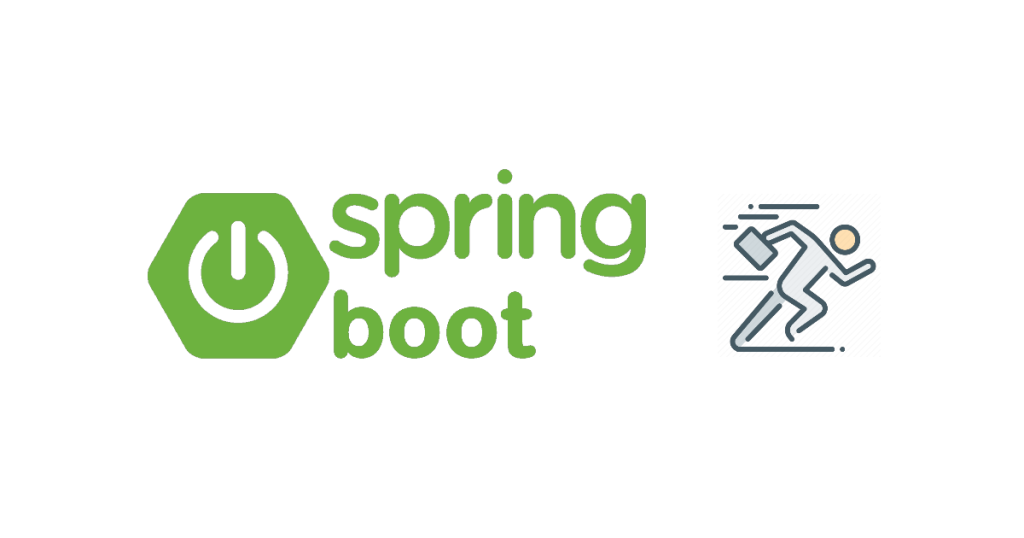
Table of Contents
Add Dependencies
Example
1. Add Dependencies
pom.xml
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
```
Create Main Application Class
2. Create Main Application Class
MainApplication.java
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MainApplication {
public static void main(String[] args) {
SpringApplication.run(MainApplication.class, args);
}
}
```
Configure Application Properties
3. Configure Application Properties
application.properties
```properties
server.port=8080
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=password
spring.jpa.database-platform=org.hibernate.dialect.H2Dialect
```
Create Configuration Classes
4. Create Configuration Classes
DatabaseConfig.java
```java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.jpa.repository.config.EnableJpaRepositories;
import org.springframework.orm.jpa.JpaTransactionManager;
import org.springframework.orm.jpa.LocalContainerEntityManagerFactoryBean;
import org.springframework.orm.jpa.vendor.HibernateJpaVendorAdapter;
import javax.persistence.EntityManagerFactory;
import javax.sql.DataSource;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.jdbc.DataSourceBuilder;
import org.springframework.transaction.PlatformTransactionManager;
@Configuration
@EnableJpaRepositories(basePackages = "com.example.repository")
public class DatabaseConfig {
@Bean
public DataSource dataSource() {
return DataSourceBuilder.create()
.url("jdbc:h2:mem:testdb")
.username("sa")
.password("password")
.driverClassName("org.h2.Driver")
.build();
}
@Bean
public LocalContainerEntityManagerFactoryBean entityManagerFactory(DataSource dataSource) {
LocalContainerEntityManagerFactoryBean emfb = new LocalContainerEntityManagerFactoryBean();
emfb.setDataSource(dataSource);
emfb.setPackagesToScan("com.example.entity");
emfb.setJpaVendorAdapter(new HibernateJpaVendorAdapter());
return emfb;
}
@Bean
public PlatformTransactionManager transactionManager(EntityManagerFactory emf) {
return new JpaTransactionManager(emf);
}
}
```
Example
5. Run the Application
User.java
```java
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String name;
// Getters and Setters
}
```
UserRepository.java
```java
import org.springframework.data.repository.CrudRepository;
public interface UserRepository extends CrudRepository<User, Long> {
}
```
UserController.java
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping("/users")
public Iterable<User> getAllUsers() {
return userRepository.findAll();
}
}
```
Summary
- Setup: Add dependencies for Spring Boot.
- Main Application Class: Use
@SpringBootApplication
andSpringApplication.run
to start the application. - Configuration: Set up application properties and configuration classes.
- Context Initialization: The application context is initialized and beans are created.
- Running the Application: The application is started and ready to handle requests.