Explain @Repository annotation example?
The `@Repository` annotation in Spring is a specialization of the `@Component` annotation. It is used to indicate that the class provides the mechanism for storage, retrieval, search, update, and delete operation on objects. The `@Repository` annotation also provides additional benefits, such as translation of database-related exceptions into Spring’s `DataAccessException` hierarchy.
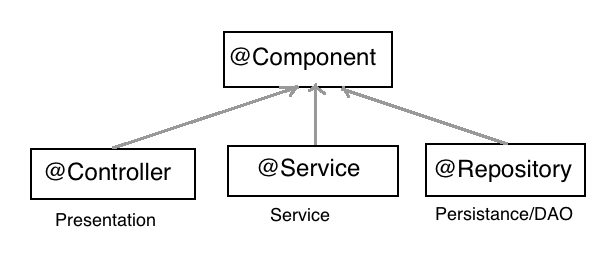
Table of Contents
Example
Step 1: Create a Spring Boot Application
Ensure you have the necessary dependencies in your `pom.xml` (for Maven) or `build.gradle` (for Gradle).
Maven Dependency:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
```
Gradle Dependency:
```groovy
implementation 'org.springframework.boot:spring-boot-starter-data-jpa'
implementation 'org.springframework.boot:spring-boot-starter-web'
runtimeOnly 'com.h2database:h2'
```
Step 2: Create the Main Application Class
Create the main class for the Spring Boot application.
```java
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
Explanation:
@SpringBootApplication
: Indicates a configuration class that declares one or more@Bean
methods and also triggers auto-configuration and component scanning.
Step 3: Create an Entity
Create a simple JPA entity.
```java
package com.example.demo.entity;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String name;
private String email;
// getters and setters
}
```
Explanation:
@Entity
: Specifies that the class is an entity and is mapped to a database table.@Id
: Specifies the primary key of an entity.@GeneratedValue
: Provides the specification of generation strategies for the values of primary keys.
Step 4: Create a Repository
Create a repository interface using the @Repository
annotation.
```java
package com.example.demo.repository;
import com.example.demo.entity.User;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
}
```
Explanation:
@Repository
: Indicates that this is a Spring Data repository.JpaRepository<User, Long>
: Provides JPA related methods such as save, findAll, findById, delete, etc.
Step 5: Create a Service
Create a service class that uses the UserRepository
.
```java
package com.example.demo.service;
import com.example.demo.entity.User;
import com.example.demo.repository.UserRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public List<User> getAllUsers() {
return userRepository.findAll();
}
public User saveUser(User user) {
return userRepository.save(user);
}
}
```
Explanation:
@Service
: Indicates that this class is a service that holds the business logic.UserRepository
: The service uses the repository to perform database operations.
Step 6: Create a Controller
Create a controller that uses the UserService
to handle web requests.
```java
package com.example.demo.controller;
import com.example.demo.entity.User;
import com.example.demo.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import java.util.List;
@Controller
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/users")
public String getUsers(Model model) {
List<User> users = userService.getAllUsers();
model.addAttribute("users", users);
return "userList";
}
}
```
Explanation:
@Controller
: Indicates that this class is a Spring MVC controller.UserService
: The controller uses the service to get data.
Step 7: Create a View
Create an HTML file named userList.html
in the src/main/resources/templates
directory.
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>User List</title>
</head>
<body>
<h1>User List</h1>
<ul>
<li th:each="user : ${users}">
<span th:text="${user.name}"></span> - <span th:text="${user.email}"></span>
</li>
</ul>
</body>
</html>
```
Explanation:
th:each
: Thymeleaf attribute for iterating over a collection.${user.name}
: Placeholder for user name.${user.email}
: Placeholder for user email.
Step 8: Running the Application
Run the application from the main class (DemoApplication
). When you navigate to http://localhost:8080/users
in your web browser, you should see a list of users.
Conclusion of Repository annotation
- @Repository: Used to indicate that the class provides the mechanism for storage, retrieval, search, update, and delete operations on objects.
- Example: Demonstrated how to use
@Repository
to create a repository interface for a JPA entity and integrate it with a service and controller.