Explain a Docker Container
A Docker container is a lightweight, standalone, and executable software package that includes everything needed to run a specific application. Containers encapsulate an application and its dependencies, such as libraries, configuration files, and environment variables, within a single package. This allows the application to run consistently across different environments, such as development, testing, and production.
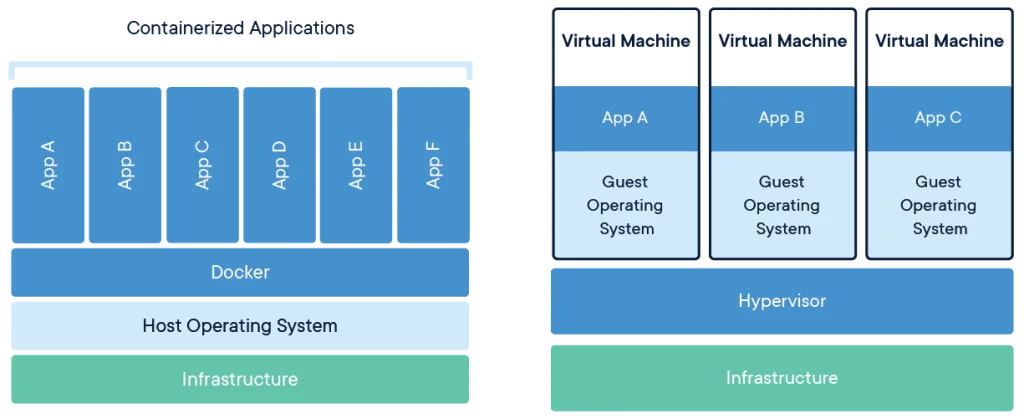
Table of Contents
Key Characteristics of a Docker Container:
1. Isolation
  Docker containers are isolated from each other and the host system. Each container runs in its own environment, with its own filesystem, process space, and network interfaces. This isolation ensures that the applications inside the containers do not interfere with each other or the host system.
2. Portability:
  Containers are portable across different environments. A containerized application can run consistently on any system that supports Docker, whether it’s a developer’s laptop, a test server, or a production cloud environment. This portability makes it easier to move applications between environments without worrying about compatibility issues.
3. Efficiency:
  Containers share the host operating system’s kernel, making them more efficient in terms of system resources compared to virtual machines. They are lightweight, start quickly, and require fewer resources (CPU, memory, and storage) because they do not need a full OS for each instance.
4. Scalability:
  Docker containers can be easily scaled up or down based on demand. Multiple instances of the same container can be run on different hosts, and containers can be managed and orchestrated using tools like Docker Swarm or Kubernetes.
5. Consistency:
  Docker containers ensure that an application runs consistently regardless of where it is deployed. The same container image can be used across different environments, eliminating the “it works on my machine” problem that often occurs in software development.
Java Example using Docker Container
Let’s demonstrate how to package and run a simple Java application in a Docker container.
1. Create a Simple Java Application:
```java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, Docker World!");
}
}
```
2. Compile the Java Application:
Compile the `HelloWorld.java` file into a `.class` file:
```sh
javac HelloWorld.java
```
3. Create a Dockerfile:
A Dockerfile is a script that contains instructions to build a Docker image.
```Dockerfile
# Use a base image with Java runtime
FROM openjdk:17-jdk-alpine
# Set the working directory inside the container
WORKDIR /app
# Copy the compiled Java class to the container
COPY HelloWorld.class /app/HelloWorld.class
# Command to run the Java application
CMD ["java", "HelloWorld"]
```
4. Build the Docker Image:
Build the Docker image from the Dockerfile:
```sh
docker build -t my-java-app .
```
This command creates a Docker image named `my-java-app`.
5. Run the Docker Container:
Run a Docker container from the image:
```sh
docker run my-java-app
```
When the container starts, it runs the Java application and outputs:
```sh
Hello, Docker World!
```
6. Stop and Remove the Container:
If needed, stop and remove the container:
```sh
docker ps # List running containers
docker stop <container_id>
docker rm <container_id>
```
Conclusion:
A Docker container provides a consistent and isolated environment for running applications. It encapsulates everything needed to run an application, making it portable, efficient, and easy to scale. Docker containers are a key component in modern DevOps practices, enabling seamless application deployment across different environments.