Explain the Components of Docker
Docker is a platform that allows developers to automate the deployment of applications in lightweight, portable containers. It includes several key components, which are:
1. Docker Engine
- The core part of Docker, responsible for creating and managing Docker containers. It is the runtime that facilitates the creation, deployment, and management of containers.
- Components of Docker Engine:
- Docker Daemon (dockerd): Runs on the host machine and listens for Docker API requests. It manages Docker objects like images, containers, networks, and volumes.
- Docker Client (docker): The command-line interface (CLI) used by users to interact with Docker. Commands entered through the Docker client are sent to the Docker daemon, which processes them.
- REST API: Docker uses REST API to interact with the Docker daemon programmatically.
2. Docker Images
- A Docker image is a lightweight, standalone, and executable package that contains everything needed to run a piece of software, including the code, runtime, libraries, environment variables, and configuration files.
- Images are read-only and serve as the basis for Docker containers.
3. Docker Containers
- A container is a runnable instance of an image. It is a lightweight, standalone, and executable software package that includes everything needed to run an application.
- Containers are isolated from each other and the host system, providing a secure and portable environment for applications.
4. Docker Registries
- A Docker registry is a storage and distribution system for Docker images. The most commonly used registry is Docker Hub, a public registry that allows users to upload and share Docker images.
- Registries can be public or private. Organizations often set up private registries to store and distribute their own images.
5. Docker Compose:
– Docker Compose is a tool that allows users to define and run multi-container Docker applications. It uses a YAML file to define the services, networks, and volumes for an application and then manages the entire application lifecycle.
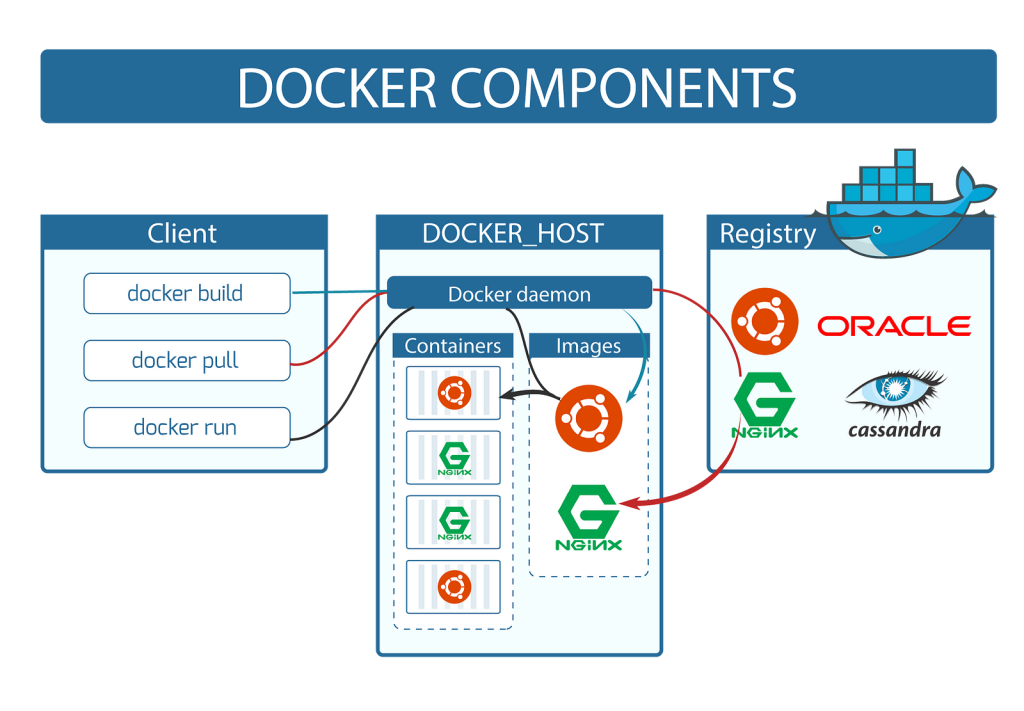
Table of Contents
6. Docker Swarm:
  Docker Swarm is a native clustering and orchestration tool for Docker. It allows you to create and manage a cluster of Docker nodes, making it easy to scale applications across multiple containers and machines.
Java Example using Components of Docker
Let’s create a simple Java application, package it as a Docker image, and run it as a Docker container.
1. Create a Simple Java Application:
```java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, Docker World!");
}
}
```
2. Create a Dockerfile:
A Dockerfile is a text document that contains instructions to build a Docker image.
```Dockerfile
Use a base image with Java runtime
FROM openjdk:17-jdk-alpine
Set the working directory inside the container
WORKDIR /app
Copy the compiled Java class to the container
COPY HelloWorld.class /app/HelloWorld.class
Command to run the Java application
CMD ["java", "HelloWorld"]
```
3. Build the Docker Image:
Run the following command in the terminal where your Dockerfile and Java class are located:
```sh
docker build -t my-java-app .
```
This command builds the Docker image and tags it as my-java-app
.
4. Run the Docker Container:
After building the image, you can run it as a Docker container:
```sh
docker run my-java-app
```
The output should be:
```sh
Hello, Docker World!
```
Components of Docker
This demonstrates how Docker can be used to package and run a simple Java application in a containerized environment