Explain @Service annotation with example?
The `@Service` annotation in Spring is a specialization of the `@Component` annotation. It is used to annotate classes that perform service tasks, typically containing the business logic. The main goal of using `@Service` is to indicate that the annotated class holds the business logic and to make it easier to find and configure services within the Spring context.
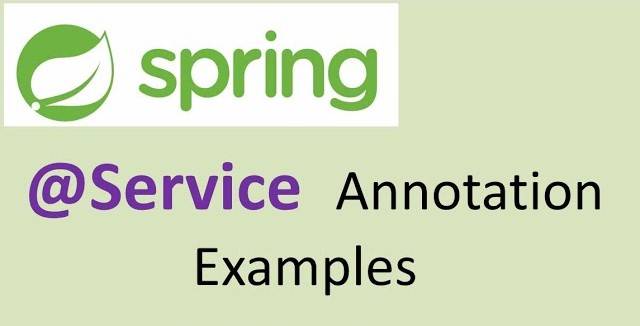
Table of Contents
Example
Step 1: Create a Spring Boot Application
Ensure you have the necessary dependencies in your `pom.xml` (for Maven) or `build.gradle` (for Gradle).
Maven Dependency:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
Gradle Dependency:
```groovy
implementation 'org.springframework.boot:spring-boot-starter-web'
```
Step 2: Create the Main Application Class
Create the main class for the Spring Boot application.
```java
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
Explanation:
@SpringBootApplication
: Indicates a configuration class that declares one or more@Bean
methods and also triggers auto-configuration and component scanning.
Step 3: Create a Service
Create a simple service class using the @Service
annotation.
```java
package com.example.demo.service;
import org.springframework.stereotype.Service;
@Service
public class GreetingService {
public String getGreeting() {
return "Hello, World!";
}
}
```
Explanation:
@Service
: Indicates that this class is a service that holds the business logic.getGreeting
: Method that returns a greeting message.
Step 4: Create a Controller
Create a controller that uses the GreetingService
to handle web requests.
```java
package com.example.demo.controller;
import com.example.demo.service.GreetingService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
@RequestMapping("/web")
public class HelloController {
private final GreetingService greetingService;
@Autowired
public HelloController(GreetingService greetingService) {
this.greetingService = greetingService;
}
@GetMapping("/hello")
public String sayHello(Model model) {
model.addAttribute("message", greetingService.getGreeting());
return "hello";
}
}
```
Explanation:
@Autowired
: Injects theGreetingService
into theHelloController
.sayHello
: Method that uses theGreetingService
to get a greeting message and adds it to the model.
Step 5: Create a View
Create an HTML file named hello.html
in the src/main/resources/templates
directory.
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello</title>
</head>
<body>
<h1>${message}</h1>
</body>
</html>
```
Explanation:
${message}
: Placeholder that will be replaced by the actual message passed from the controller.
Step 6: Running the Application
Run the application from the main class (DemoApplication
). When you navigate to http://localhost:8080/web/hello
in your web browser, you should see the message: “Hello, World!” displayed on the page.
Conclusion of Service annotation
- @Service: Used to define a service that holds the business logic in a Spring application.
- Example: Demonstrated how to use
@Service
to create a simple service class and integrate it with a controller to display a greeting message.