EnableAutoConfiguration annotation
The `@EnableAutoConfiguration` annotation is a key feature of Spring Boot that enables automatic configuration of the Spring application context. When Spring Boot starts, it tries to automatically configure your application based on the jar dependencies you have added. It scans the classpath for beans and registers them based on the dependencies and properties you have defined.
The main purpose of `@EnableAutoConfiguration` is to reduce the need for explicit configuration in the application, making it Explain easier to get started with Spring applications.
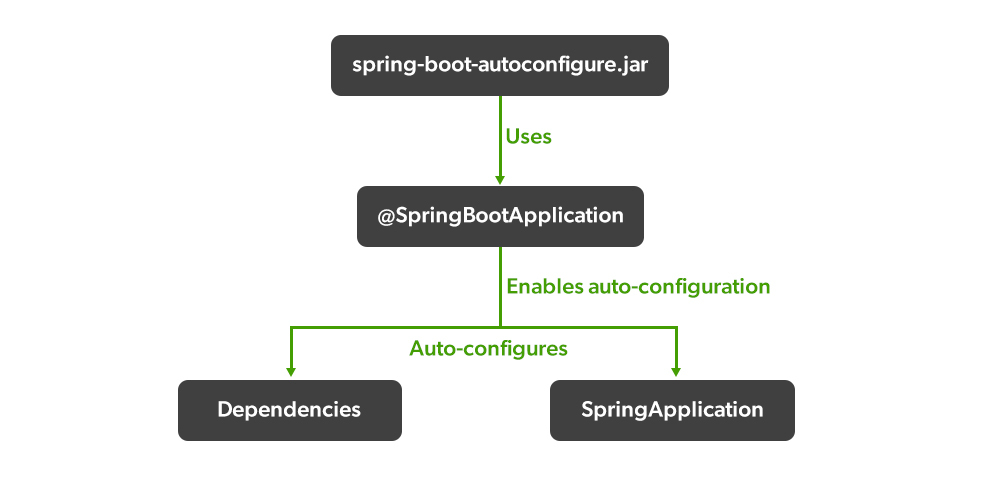
Table of Contents
Example
Step 1: Create a Spring Boot Application
Create a simple Spring Boot application that uses the `@EnableAutoConfiguration` annotation
Step 2: Add Dependency
Ensure you have the necessary dependencies in your pom.xml
(for Maven) or build.gradle
(for Gradle).
Maven Dependency:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
```
Gradle Dependency:
```groovy
implementation 'org.springframework.boot:spring-boot-starter'
```
Step 3: Create the Main Application Class
Create the main class with the @EnableAutoConfiguration
annotation.
```java
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.context.annotation.ComponentScan;
@EnableAutoConfiguration
@ComponentScan(basePackages = "com.example.demo")
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
Explanation:
@EnableAutoConfiguration
: Tells Spring Boot to start adding beans based on classpath settings, other beans, and various property settings.@ComponentScan(basePackages = "com.example.demo")
: Specifies the base package to scan for Spring components.
Step 4: Create a REST Controller
Create a simple REST controller to handle HTTP requests.
```java
package com.example.demo.controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, World!";
}
}
```
Explanation:
@RestController
: Indicates that this class is a REST controller.@GetMapping("/hello")
: Maps the/hello
URL to thesayHello
method.
Step 5: Running the Application
Run the application from the main class (DemoApplication
). When you navigate to http://localhost:8080/hello
in your web browser, you should see the message: “Hello, World!”.
Conclusion of EnableAutoConfiguration annotation
- @EnableAutoConfiguration: Automatically configures the Spring application context based on classpath settings, beans, and properties.
- Example: Demonstrated how to use
@EnableAutoConfiguration
to create a simple Spring Boot application with a REST controller.