PathVariable annotation
The `@PathVariable` annotation in Spring is used to extract values from the URI path of a request and bind them to method parameters in a controller. This is especially useful when you need to capture values from the URL itself, such as IDs or other parameters, which are often used to identify specific resources.
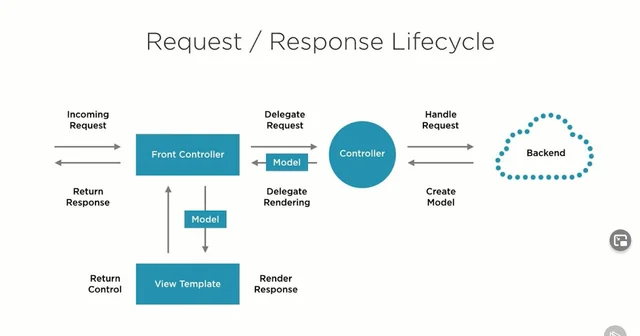
Table of Contents
Example
- Step 1: Create a Spring Boot Application
- Ensure you have the necessary dependencies in your `pom.xml` (for Maven) or `build.gradle` (for Gradle).
Maven Dependency
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
Gradle Dependency
```groovy
implementation 'org.springframework.boot:spring-boot-starter-web'
```
- Step 2: Create the Main Application Class
- Create the main class for the Spring Boot application.
Example
```java
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
Explanation:
- `@SpringBootApplication`: Indicates a configuration class that declares one or more `@Bean` methods and also triggers auto-configuration and component scanning.
- Step 3: Create a Controller
- Create a controller class that uses the `@PathVariable` annotation to handle incoming requests with path variables.
Example
```java
package com.example.demo.controller;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api")
public class UserController {
@GetMapping("/users/{id}")
public String getUserById(@PathVariable("id") Long userId) {
return "User ID: " + userId;
}
@GetMapping("/users/{id}/name/{name}")
public String getUserByIdAndName(@PathVariable("id") Long userId, @PathVariable("name") String userName) {
return "User ID: " + userId + ", Name: " + userName;
}
}
```
Explanation:
- `@RestController`: Indicates that this class is a REST controller, and the return values of methods are directly written to the HTTP response body.
- `@RequestMapping(“/api”)`: Specifies that all methods in this class handle requests starting with `/api`.
- `@GetMapping(“/users/{id}”)`: Maps GET requests to `/api/users/{id}` to the `getUserById` method. `{id}` is a path variable that will be extracted from the URL.
- `@PathVariable(“id”)`: Binds the `{id}` path variable from the URL to the `userId` method parameter.
- `@GetMapping(“/users/{id}/name/{name}”)`: Maps GET requests to `/api/users/{id}/name/{name}` to the `getUserByIdAndName` method. `{id}` and `{name}` are path variables that will be extracted from the URL.
- `@PathVariable(“name”)`: Binds the `{name}` path variable from the URL to the `userName` method parameter.
- Step 4: Running the Application
- Run the application from the main class (`DemoApplication`). You can test the endpoints using a tool like Postman or by navigating to the URLs in your browser:
- GET request to `http://localhost:8080/api/users/123` should return `”User ID: 123″`.
- GET request to `http://localhost:8080/api/users/123/name/John` should return `”User ID: 123, Name: John”`.
Conclusion
- `@PathVariable`: Used to extract values from the URI path and bind them to method parameters. It is useful for capturing dynamic values from the URL.
- Example: Demonstrated how to use `@PathVariable` to handle requests with path variables and extract them into method parameters.