Qualifier annotation with example
The `@Qualifier` annotation in Spring is used to resolve ambiguity when multiple beans of the same type are present in the Spring application context. It allows you to specify which bean should be injected when there are multiple candidates. This is particularly useful in dependency injection scenarios where you have several implementations of an interface and need to indicate which one should be used.
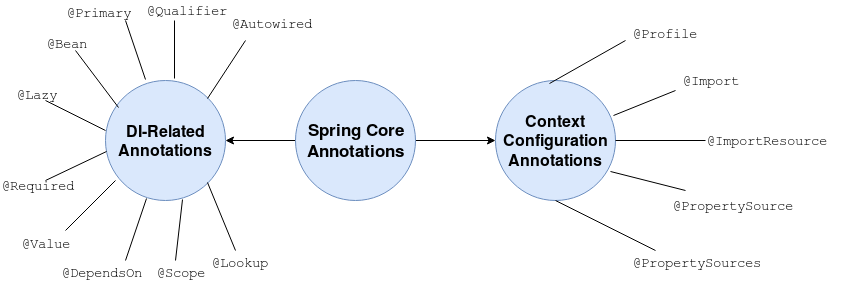
Table of Contents
Example
- Step 1: Create a Spring Boot Application
- Ensure you have the necessary dependencies in your `pom.xml` (for Maven) or `build.gradle` (for Gradle).
Maven Dependency
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
```
Gradle Dependency
```groovy
implementation 'org.springframework.boot:spring-boot-starter'
```
- Step 2: Create the Main Application Class
- Create the main class for the Spring Boot application.
Example
```java
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
Explanation:
- `@SpringBootApplication`: Indicates a configuration class that declares one or more `@Bean` methods and also triggers auto-configuration and component scanning.
- Step 3: Define a Common Interface
- Define an interface that will be implemented by multiple classes.
Example
```java
package com.example.demo.service;
public interface GreetingService {
String greet();
}
```
Explanation:
- `GreetingService`: An interface with a method `greet` to be implemented by different classes.
- Step 4: Implement Multiple Beans
- Create multiple implementations of the `GreetingService` interface.
Example
```java
package com.example.demo.service;
import org.springframework.stereotype.Service;
@Service("englishGreetingService")
public class EnglishGreetingService implements GreetingService {
@Override
public String greet() {
return "Hello!";
}
}
@Service("spanishGreetingService")
public class SpanishGreetingService implements GreetingService {
@Override
public String greet() {
return "¡Hola!";
}
}
```
Explanation:
- `@Service`: Indicates that a class is a Spring service. This annotation also makes the class eligible for component scanning.
- `@Service(“englishGreetingService”)`: Registers `EnglishGreetingService` with the name “englishGreetingService”.
- `@Service(“spanishGreetingService”)`: Registers `SpanishGreetingService` with the name “spanishGreetingService”.
- Step 5: Use @Qualifier in Dependency Injection
- Inject a specific implementation of `GreetingService` using `@Qualifier`.
Example
```java
package com.example.demo.controller;
import com.example.demo.service.GreetingService;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/greet")
public class GreetingController {
private final GreetingService greetingService;
public GreetingController(@Qualifier("englishGreetingService") GreetingService greetingService) {
this.greetingService = greetingService;
}
@GetMapping
public String greet() {
return greetingService.greet();
}
}
```
Explanation:
- `@Qualifier(“englishGreetingService”)`: Specifies that the `GreetingService` bean to be injected is the one with the name “englishGreetingService”.
- `GreetingController`: A REST controller that uses dependency injection to get a `GreetingService` implementation. It uses the `@Qualifier` annotation to choose the specific implementation.
- Step 6: Running the Application
- Run the application from the main class (`DemoApplication`). You can test the endpoint using a tool like Postman or by navigating to the URL in your browser:
GET request to `http://localhost:8080/greet` should return `”Hello!”` as the response because the `EnglishGreetingService` is injected.
Conclusion:
- `@Qualifier`: Used to resolve ambiguity when multiple beans of the same type are present. It specifies which bean should be injected in cases where multiple candidates are available.
- Example: Demonstrated how to use `@Qualifier` to specify which bean to inject when there are multiple implementations of an interface.