servlets are accessible user a valid session
To ensure that all servlets in your web application are accessible only when the user has a valid session, you can implement a session validation mechanism. This can be achieved using servlet filters, which allow you to intercept requests before they reach.
By creating a filter that checks for a valid session, you can redirect users to a login page if they are not authenticated or if their session has expired. This centralizes the session validation logic, making your application easier to maintain and secure.
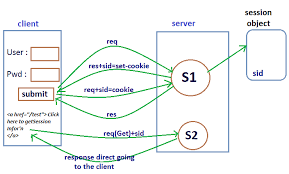
Table of Contents
Steps to Implement Session Validation
1. Â Create a Session Validation Filter
- This filter will check if a user session exists and is valid.
- If the session is invalid, the filter will redirect the user to a login page.
2. Â Configure the Filter in web.xml
  Map the filter to the URLs you want to protect. Typically, this would be all URLs except the login and public pages.
Step 1: Create the Session Validation Filter
java
import java.io.IOException;
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.FilterConfig;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
public class SessionValidationFilter implements Filter {
@Override
public void init(FilterConfig filterConfig) throws ServletException {
// Initialization code, if needed
}
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException {
HttpServletRequest httpRequest = (HttpServletRequest) request;
HttpServletResponse httpResponse = (HttpServletResponse) response;
HttpSession session = httpRequest.getSession(false);
// Check if session exists and user is logged in
if (session == null || session.getAttribute("user") == null) {
// No valid session, redirect to login page
httpResponse.sendRedirect(httpRequest.getContextPath() + "/login.jsp");
} else {
// Valid session, continue to the requested resource
chain.doFilter(request, response);
}
}
@Override
public void destroy() {
// Cleanup code, if needed
}
}
Step 2: Configure the Filter in web.xml
xml
<filter>
<filter-name>SessionValidationFilter</filter-name>
<filter-class>com.example.SessionValidationFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>SessionValidationFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
Summary
- Session Validation Filter : Intercepts all incoming requests to check for a valid session.
- Redirect to Login Page : If the session is invalid or does not exist, redirect the user to the login page.
- Centralized Security : By using a filter, you centralize session validation logic, making your application more secure and maintainable.
Considerations
- Login and Public Pages : Make sure to exclude login and other public pages from the filter. You can add additional logic in the filter to allow access to these pages without a session.
- User Object : The example assumes a “user” attribute in the session, which would be set upon successful login.