Key Steps for File Upload
Struts2 provides built-in support for file uploads through the FileUploadInterceptor interceptor. This interceptor handles the file upload process, including setting properties on your action class, validating file types and sizes, and saving the file to a specified location.
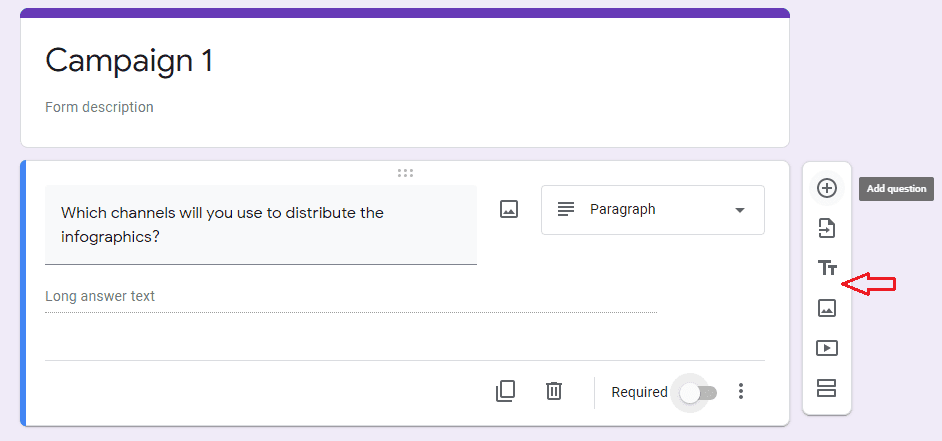
Table of Contents
Key Steps for File Upload:
- 1. Â Configure FileUploadInterceptor : Add the FileUploadInterceptor to your action configuration.
- 2. Â Create Action Class : Define properties in your action class to receive the uploaded file and related metadata.
- 3. Â Configure JSP Form : Create a JSP form that uses the multipart/form-data encoding type to allow file uploads.
- 4. Â Define Struts Configuration : Configure the action and result in struts.xml.
Java Example
Let’s create a simple Struts2 application that allows users to upload a file.
Step 1: Configure Struts2 for File Upload
xml
<!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<package name="default" namespace="/" extends="struts-default">
<!-- Configure the action for file upload -->
<action name="uploadFile" class="com.example.action.FileUploadAction">
<interceptor-ref name="defaultStack">
<!-- Add FileUploadInterceptor to the interceptor stack -->
<interceptor-ref name="fileUpload"/>
</interceptor-ref>
<result name="input">/fileUpload.jsp</result>
<result name="success">/uploadSuccess.jsp</result>
</action>
</package>
</struts>
Step 2: Create Struts2 Action for File Upload
java
package com.example.action;
import com.opensymphony.xwork2.ActionSupport;
import org.apache.commons.io.FileUtils;
import java.io.File;
import java.io.IOException;
public class FileUploadAction extends ActionSupport {
private File upload;
private String uploadContentType;
private String uploadFileName;
private String destPath;
@Override
public String execute() {
destPath = "C:/uploads/"; // Destination directory
try {
File destFile = new File(destPath, uploadFileName);
FileUtils.copyFile(upload, destFile);
} catch (IOException e) {
e.printStackTrace();
return ERROR;
}
return SUCCESS;
}
public File getUpload() {
return upload;
}
public void setUpload(File upload) {
this.upload = upload;
}
public String getUploadContentType() {
return uploadContentType;
}
public void setUploadContentType(String uploadContentType) {
this.uploadContentType = uploadContentType;
}
public String getUploadFileName() {
return uploadFileName;
}
public void setUploadFileName(String uploadFileName) {
this.uploadFileName = uploadFileName;
}
}
Step 3: Create JSP Form for File Upload
jsp
<%@ taglib uri="/struts-tags" prefix="s" %>
<html>
<head>
<title>File Upload</title>
</head>
<body>
<h2>Upload a File</h2>
<s:form action="uploadFile" method="post" enctype="multipart/form-data">
<s:file name="upload" label="Select a File"/>
<s:submit value="Upload"/>
</s:form>
</body>
</html>
Step 4: Create JSP Page for Successful Upload
jsp
<html>
<head>
<title>Upload Success</title>
</head>
<body>
<h2>File uploaded successfully!</h2>
</body>
</html>
Explanation of the Example
- 1. Â Configuration : The struts.xml file configures the action uploadFile with the FileUploadInterceptor added to the interceptor stack.
- 2. Â Struts2 Action : FileUploadAction.java defines properties for the uploaded file, its content type, and file name. The execute method handles saving the file to a specified directory.
- 3. Â JSP Form : fileUpload.jsp contains a form with multipart/form-data encoding to allow file uploads. The form includes a file input field and a submit button.
- 4. Â Success Page : uploadSuccess.jsp is displayed after a successful file upload.
Benefits:
- Ease of Use : Struts2’s FileUploadInterceptor simplifies the process of handling file uploads.
- Validation : The interceptor can automatically validate file size and type.
- Configuration : Easily configurable through struts.xml and JSP.
Testing the File Upload
To test the file upload functionality:
- 1. Deploy the application on your server.
- 2. Navigate to http://localhost:8080/yourapp/fileUpload.jsp.
- 3. Select a file and click the upload button.
- 4. Verify that the file is uploaded to the specified directory and that the success page is displayed.